Backup-SqlDatabase -ServerInstance SQL-01 -Database Whatever -BackupFile "C:\WhereEver\Backup\Test.bak" -Verbose
SQL Bring Database Online
USE [master]
GO
ALTER DATABASE [database name] SET ONLINE
GO
Find Source of AD Account Lockout
<#
.SYNOPSIS
This function locates the computer that processed a failed user logon attempt which caused a user account to become locked out.
.DESCRIPTION
This function will locate the computer that processed a failed user logon attempt which caused the user account to become locked out.
The locked out location is found by querying the PDC Emulator for locked out events (4740).
The function will display the BadPasswordTime attribute on all of the domain controllers to add in further troubleshooting.
.EXAMPLE
PS C:\>Get-LockedOutLocation -Identity Jimmy.John
This example will find the locked out location for Jimmy John.
.NOTE
-This function is only compatible with an environment where the domain controller with the PDC Emulator role is running Windows Server 2008 SP2 and higher.
-The script is dependent on the ActiveDirectory PowerShell module, which requires the AD Web services to be running on at least one domain controller.
Author: Brandon Lanczak
Last Modified: 10-08-2022 @ 21:00 CST
#>
Function Get-LockedOutLocation {
[CmdletBinding()]
Param(
[Parameter(Mandatory=$True)]
[String]$Identity
)
Begin {
$DCCounter = 0
$LockedOutStats = @()
Try { Import-Module ActiveDirectory -ErrorAction Stop }
Catch {
Write-Warning $_
Break
}
}
Process {
#Get all domain controllers in domain
$DomainControllers = Get-ADDomainController -Filter *
$PDCEmulator = $DomainControllers | Where-Object { $_.OperationMasterRoles -contains "PDCEmulator" }
Write-Verbose "Finding the domain controllers in the domain"
ForEach($DC in $DomainControllers) {
$DCCounter++
Write-Progress -Activity "Contacting DCs for lockout info" -Status "Querying $($DC.Hostname)" -PercentComplete (($DCCounter/$DomainControllers.Count) * 100)
Try { $UserInfo = Get-ADUser -Identity $Identity -Server $DC.Hostname -Properties AccountLockoutTime,LastBadPasswordAttempt,BadPwdCount,LockedOut -ErrorAction Stop }
Catch {
Write-Warning $_
Continue
}
if ($UserInfo.LastBadPasswordAttempt) {
$LockedOutStats += New-Object -TypeName PSObject -Property @{
Name = $UserInfo.SamAccountName
SID = $UserInfo.SID.Value
LockedOut = $UserInfo.LockedOut
BadPwdCount = $UserInfo.BadPwdCount
BadPasswordTime = $UserInfo.BadPasswordTime
DomainController = $DC.Hostname
AccountLockoutTime = $UserInfo.AccountLockoutTime
LastBadPasswordAttempt = ($UserInfo.LastBadPasswordAttempt).ToLocalTime()
}
}
}
$LockedOutStats | Format-Table -Property Name,LockedOut,DomainController,BadPwdCount,AccountLockoutTime,LastBadPasswordAttempt -AutoSize
#Get User Info
Try {
Write-Verbose "Querying event log on $($PDCEmulator.HostName)"
$LockedOutEvents = Get-WinEvent -ComputerName $PDCEmulator.HostName -FilterHashtable @{LogName='Security';Id=4740} -ErrorAction Stop | Sort-Object -Property TimeCreated -Descending
}
Catch {
Write-Warning $_
Continue
}
ForEach ($Event in $LockedOutEvents) {
if ($Event | Where {$_.Properties[2].value -match $UserInfo.SID.Value}) {
$Event | Select-Object -Property @(
@{Label = 'User'; Expression = {$_.Properties[0].Value}}
@{Label = 'DomainController'; Expression = {$_.MachineName}}
@{Label = 'EventId'; Expression = {$_.Id}}
@{Label = 'LockedOutTimeStamp'; Expression = {$_.TimeCreated}}
@{Label = 'Message'; Expression = {$_.Message -split "`r" | Select -First 1}}
@{Label = 'LockedOutLocation'; Expression = {$_.Properties[1].Value}}
)
}
}
}
}
Sheep
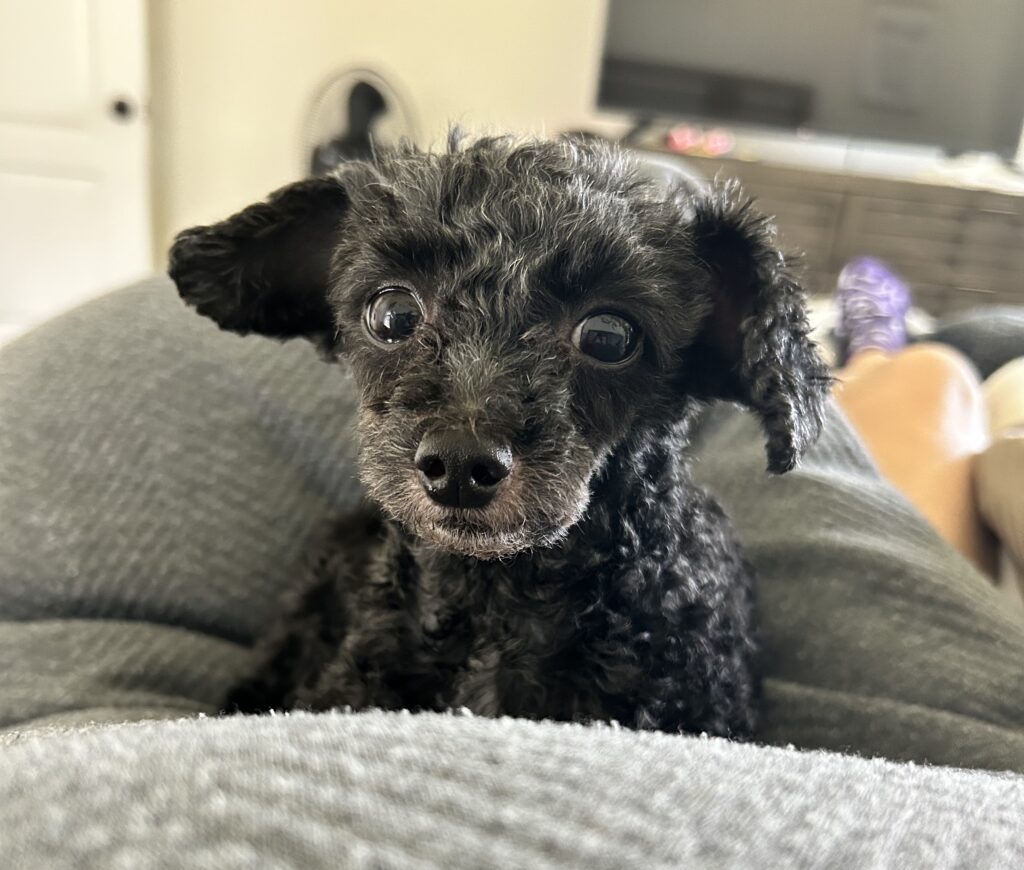
A normal day
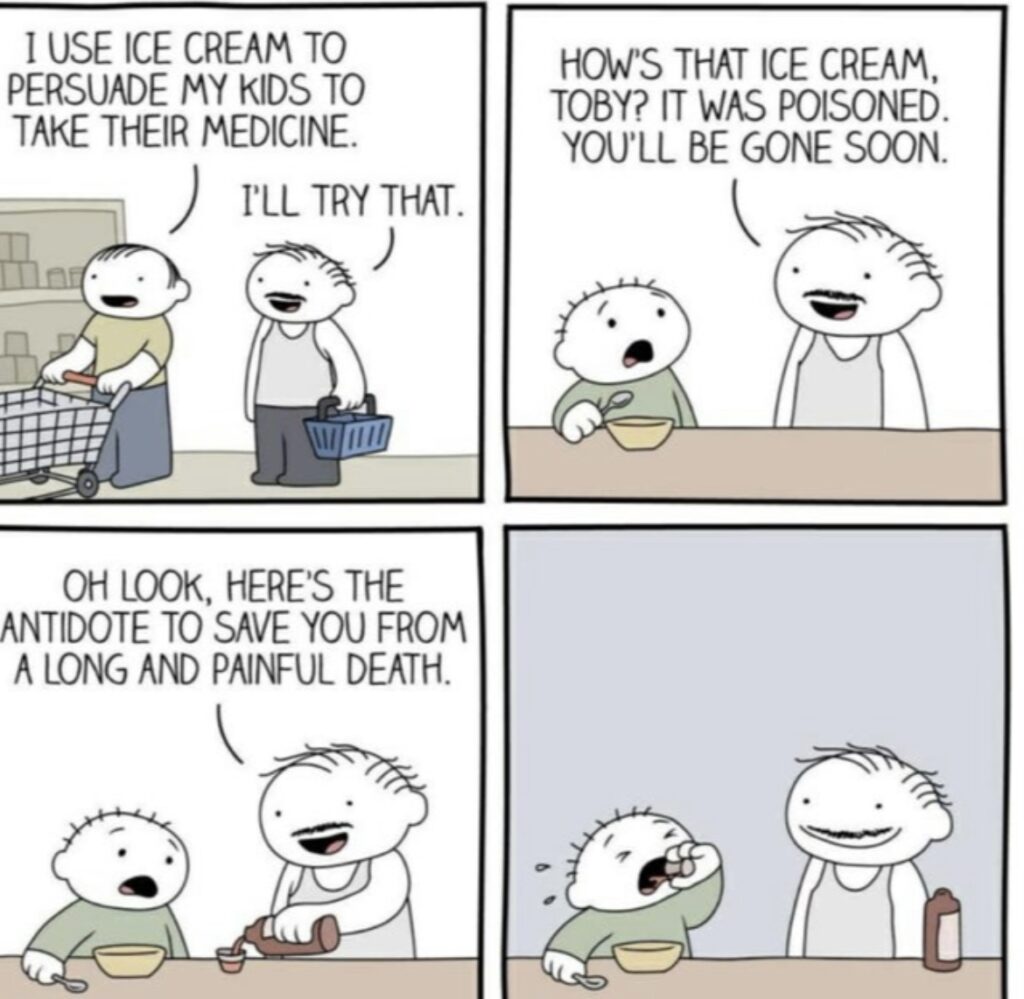
PowerShell Flush DNS on multiple computers
# Flush DNS on multiple computers
$Servers = "Server01","Server02","Server03"
foreach ($Server in $Servers) {
Invoke-WmiMethod -Class Win32_Process -Name Create -ArgumentList ("cmd.exe /c ipconfig /flushdns") -ComputerName $Server
Invoke-WmiMethod -Class Win32_Process -Name Create -ArgumentList ("cmd.exe /c ipconfig /registerdns") -ComputerName $Server
}
Simple PowerShell Countdown Timer
[int]$Time = Read-Host "Enter time in minutes"
$Time = $Time * 60
$Length = $Time / 100
For ($Time; $Time -gt 0; $Time--) {
$min = [int](([string]($Time/60)).split('.')[0])
$Text = " " + $min + " minutes " + ($Time % 60) + "seconds left"
Write-Progress -Activity "Waiting for..." -Status $Text -PercentComplete ($Time / $Length)
Start-Sleep 1
}
Python Port Scanner
from datetime import datetime
# Clear the screen
subprocess.call('clear', shell=True)
# Ask for input
remoteServer = raw_input("Enter a remote host to scan: ")
remoteServerIP = socket.gethostbyname(remoteServer)
# Print a nice banner with information on which host we are about to scan
print "-" * 60
print "Please wait, scanning remote host", remoteServerIP
print "-" * 60
# Check what time the scan started
t1 = datetime.now()
# Using the range function to specify ports (here it will scans all ports between 1 and 1024)
# We also put in some error handling for catching errors
try:
for port in range(1,1025):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
result = sock.connect_ex((remoteServerIP, port))
if result == 0:
print "Port {}: Open".format(port)
sock.close()
except KeyboardInterrupt:
print "You pressed Ctrl+C"
sys.exit()
except socket.gaierror:
print 'Hostname could not be resolved. Exiting'
sys.exit()
except socket.error:
print "Couldn't connect to server"
sys.exit()
# Checking the time again
t2 = datetime.now()
# Calculates the difference of time, to see how long it took to run the script
total = t2 - t1
# Printing the information to screen
print 'Scanning Completed in: ', total
Extract IP Addresses from File with PowerShell
$input_path = ‘c:\temp\input_file.txt’
$output_file = ‘c:\temp\extracted_ip_addresses.txt’
$regex = ‘\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b’
select-string -Path $input_path -Pattern $regex -AllMatches | % { $_.Matches } | % { $_.Value } > $output_file
I’m back
Might give this a try again.