# Specify the root folder path
$rootFolderPath = "C:\Temp"
# Get all items (files and subfolders) under the root folder
$items = Get-ChildItem -Path $rootFolderPath -Recurse
# Iterate through each item
foreach ($item in $items) {
# Get the ACL for the item
$acl = Get-Acl -Path $item.FullName
# Check if any explicit permissions exist (not inherited)
$explicitPermissions = $acl.Access | Where-Object { $_.IsInherited -eq $false }
if ($explicitPermissions.Count -gt 0) {
Write-Host "Explicit permissions found for $($item.FullName):"
foreach ($permission in $explicitPermissions) {
Write-Host " User: $($permission.IdentityReference)"
Write-Host " Permissions: $($permission.FileSystemRights)"
Write-Host " Access Control Type: $($permission.AccessControlType)"
Write-Host " Inherited: $($permission.IsInherited)"
Write-Host ""
}
}
}
Validate SHA256 Checksums with Python
import tkinter as tk
from tkinter import filedialog, simpledialog, messagebox
import hashlib
def calculate_sha256(file_path):
sha256_hash = hashlib.sha256()
with open(file_path, "rb") as f:
# Read and update hash string value in blocks of 4K
for byte_block in iter(lambda: f.read(4096), b""):
sha256_hash.update(byte_block)
return sha256_hash.hexdigest()
def verify_checksum():
file_path = filedialog.askopenfilename(title="Select the file to check the SHA256 checksum on", filetypes=[("All Files", "*.*")])
if file_path:
# Prompt user for SHA256 checksum
entered_checksum = simpledialog.askstring("Checksum Verification", "Enter SHA256 checksum:")
# Calculate the SHA256 checksum of the selected file
file_checksum = calculate_sha256(file_path)
# Compare entered checksum with calculated checksum
if entered_checksum == file_checksum:
messagebox.showinfo("Verification Result", "Checksum verification successful. The file has not been tampered with.")
else:
messagebox.showerror("Verification Result", "Checksum verification failed. The file may have been tampered with.")
else:
messagebox.showinfo("File Selection", "File selection cancelled by the user.")
# Create the main application window
root = tk.Tk()
root.withdraw() # Hide the main window
# Call the verify_checksum function
verify_checksum()
# Start the main loop
root.mainloop()
Don’t Scan Random QR codes
Don’t scan codes from strangers (if you receive a QR code from an unsolicited message — text, social media, email, or physical — be skeptical and don’t use it!) If it’s a physical QR code — take a look to see if it was tampered with.
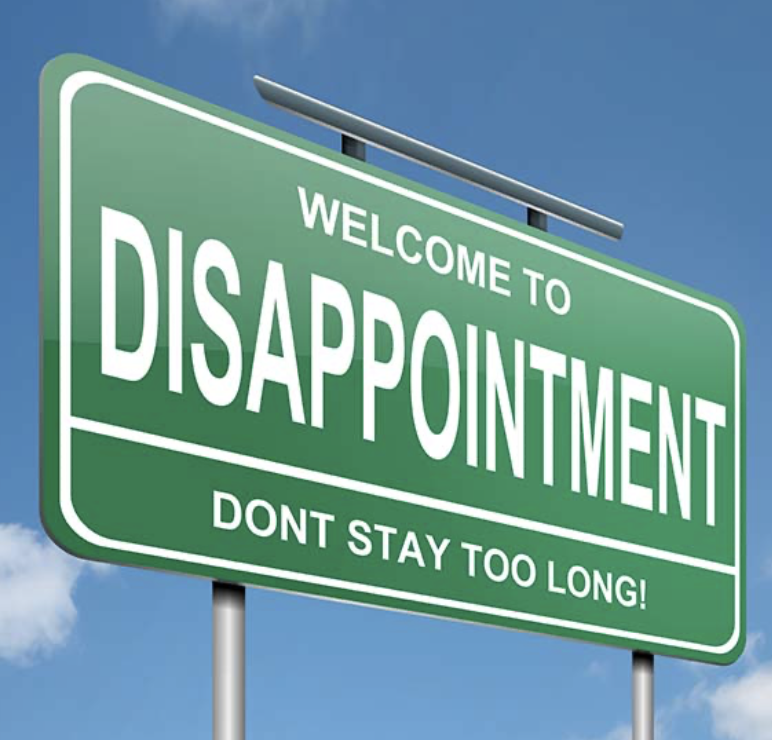
Ransomware gang files SEC complaint over victim’s undisclosed breach
Check service status on multiple nodes
# List of remote computer names
$computers = @("Server01", "Server02", "Server03", "Server04", "Server05", "Server06")
# Name of the service you want to check
$serviceName = "PrintSpooler"
# Loop through the list of remote computers and check the service status
foreach ($computer in $computers) {
try {
$serviceStatus = Get-Service -ComputerName $computer -Name $serviceName
Write-Host "Service $serviceName on $computer is $($serviceStatus.Status)"
} catch {
Write-Host "Failed to get service status on" $computer
}
}
Show all users RDP’d into many computers
# List of computer names or IP addresses.
# Note: Un-comment the preferred source
#$computers = @("ServerA", "ServerB")
#$computers = Get-Content -Path "C:\TEMP\AllNodes.txt
foreach ($computer in $computers) {
Write-Host "Users logged on to"$computer":"
try {
$loggedOnUsers = query user /server:$computer 2>&1 | ForEach-Object {
# Split the line into individual pieces of information
$userDetails = $_ -split '\s+'
if ($userDetails[1] -ne "USERNAME" -and $userDetails[1] -ne "No") {
# Filter out header and empty lines
$userDetails[1]
}
}
if ($loggedOnUsers -eq $null) {
Write-Host "No users are currently logged on to $computer."
} else {
$loggedOnUsers
}
} catch {
Write-Host "Error connecting to "$computer": $_"
}
Write-Host
}
Check for Windows Activation for a group of nodes
# Define list of nodes to check
$servers = Get-Content -Path "C:\TEMP\AllNodes.txt"
# Query activation status
foreach ($server in $servers) {
$activation = Get-WmiObject -Query "SELECT * FROM SoftwareLicensingProduct WHERE (PartialProductKey IS NOT NULL)" -ComputerName $server
if ($activation) {
Write-Host "Server $server is activated."
} else {
Write-Host "Server $server is not activated."
}
}
SolarWinds File Rate of Growth Monitor
# This script can be used with SolarWinds SAM to monitor for rate of growth for a specific file
# Globally used variables
$FileToMonitor = "C:\Temp\FileToMonitor.txt"
$CacheFile = "C:\Temp\Cache.txt"
# Get Current file size
$FileSize = Get-Item -Path $FileToMonitor | Select-Object -ExpandProperty Length
# Write current file size to a cache file
Add-Content $CacheFile $FileSize
# Calculate the percent difference and round it to a whole number
$oldValue = Get-Content $CacheFile -First 1
$newValue = Get-Content $CacheFile -Last 1
$percentageDifference = (($newValue - $oldValue) / $oldValue) * 100
$percentageDifference = ([Math]::Round($percentageDifference, 0))
# Write to the host for SolarWinds to ingest
Write-Host "Message: File growth was calculated as $percentageDifference%"
Write-Host "Statistic:" $percentageDifference
# Keep the cache file clean/small (litterally just keeping 2-lines)
(Get-Content "$CacheFile" -Tail 2) | Set-Content "$CacheFile"
# Exit Session
Exit 0;
PowerShell Append 2mb of Junk to a .txt file
# Specify the path to the existing text file
$filePath = "C:\TEMP\Rubbish.txt"
# Calculate the size of 2MB in bytes
$additionalDataSize = 2 * 1024 * 1024
# Generate 2MB of random data
$randomText = [byte[]]::new($additionalDataSize)
$random = New-Object System.Random
$random.NextBytes($randomText)
# Open the file in append mode and write the random data
$fileStream = [System.IO.File]::Open($filePath, [System.IO.FileMode]::Append)
$fileStream.Write($randomText, 0, $randomText.Length)
$fileStream.Close()
Write-Host "Added 2MB of random data to $filePath"
Encode Files with Certutil
Encode
certutil -encode "C:\Wherever\Whatever.whatever" "C:\Wherever.whatever"
Decode
certutil -decode "C:\Wherever\Whatever.whatver" "C:\Wherever\Whatever.Whatever"